pyfast_and_pyside2.py source
This example demonstrates how to use FAST together with Qt Python GUI with PySide2.
This example demonstrates how to use FAST together with Qt Python GUI with PySide2
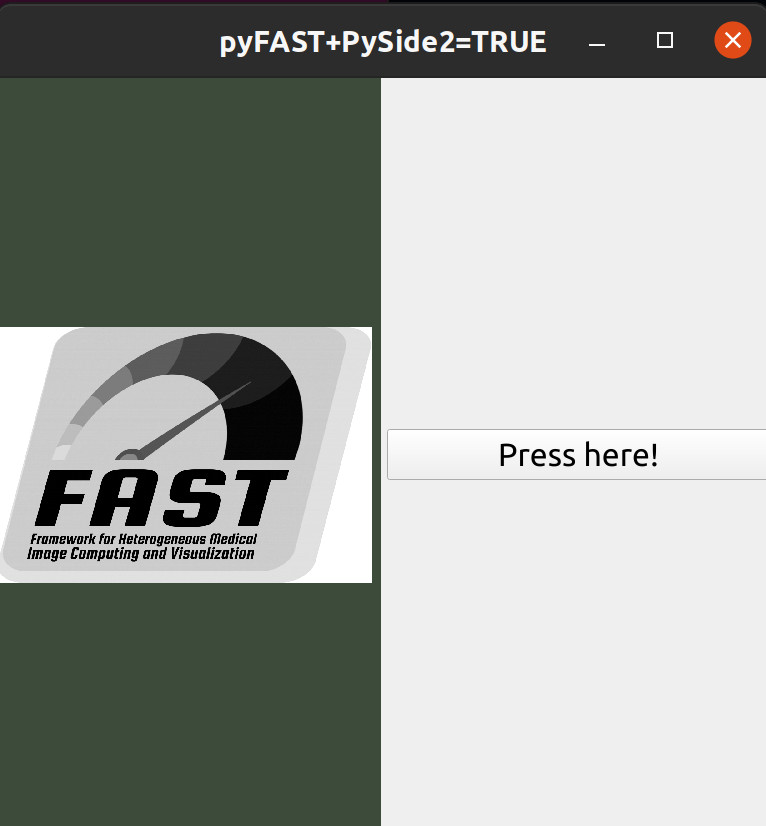
import platform if platform.system() != 'Windows': import PySide2.QtSvg # Must import this before fast due to conflicting symbols import fast # Must import FAST before rest of pyside2 from PySide2.QtWidgets import * from shiboken2 import wrapInstance from random import random # Setup a FAST pipeline importer = fast.ImageFileImporter\ .create(fast.Config.getDocumentationPath() + '/images/FAST_logo_square.png') renderer = fast.ImageRenderer.create()\ .connect(importer) window = fast.SimpleWindow2D.create()\ .connect(renderer) window.setTitle('pyFAST+PySide2=TRUE') # Add Qt button to the FAST window button = QPushButton("Press here!") # Convert FAST QWidget pointer to a PySide2 QWidget mainWidget = wrapInstance(int(window.getWidget()), QWidget) layout = mainWidget.layout() layout.addWidget(button) # Add event: When button is clicked, change background color of the FAST view randomly button.clicked.connect(lambda: window.getView().setBackgroundColor(fast.Color(random(), random(), random()))) # Run everything! window.run()